SolidJS vs Svelte vs Astro Feature Analysis of Web Frameworks
Compare SolidJS, Svelte and Astro frameworks with detailed analysis of features and performance to help you choose the right solution for your needs.

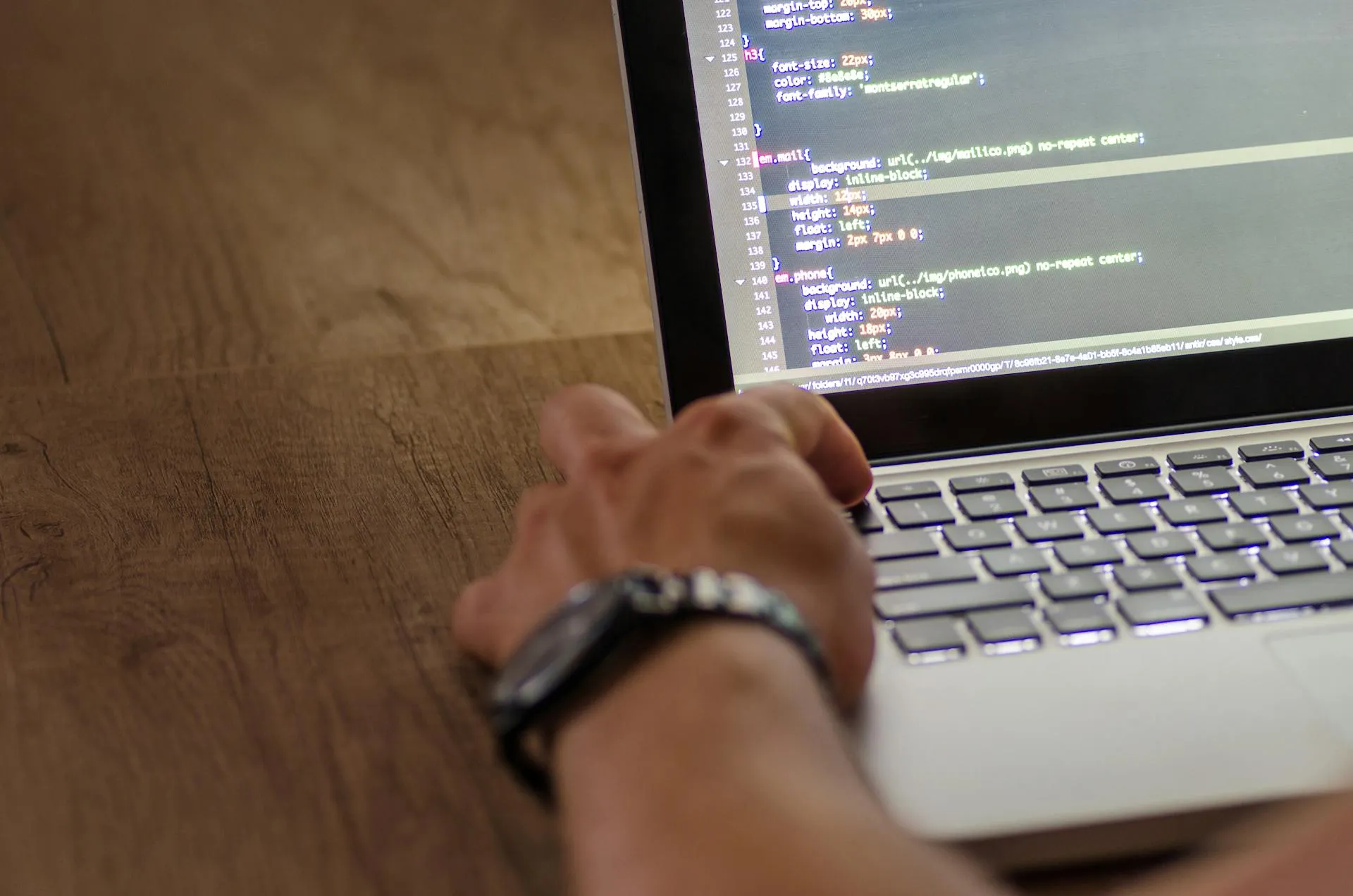
Choosing the proper framework in today’s web development landscape can significantly impact your project’s success. As businesses increasingly prioritise performance and user experience, lightweight frameworks have gained substantial attention. Let’s dive into a comprehensive comparison of three popular contenders: SolidJS, Svelte, and Astro.
Table of Contents
- Table of Contents
- Understanding Lightweight Frontend Frameworks
- Meet the Contenders
- SolidJS: Ultra-Fine-Grained Reactivity
- Svelte: The Compiler-First Framework
- Astro: The All-in-One Web Framework
- Key Features Comparison
- Making the Right Choice for Your Project
- Strategic Framework Selection
- Professional Guidance and Support
- Conclusion: A Framework for Every Need
Understanding Lightweight Frontend Frameworks
Lightweight frontend frameworks are JavaScript libraries that help developers build web applications with minimal overhead. These frameworks aim to deliver exceptional performance by reducing bundle sizes and optimising runtime execution. This approach leads to faster load times, better user experience, and improved search engine rankings.
Meet the Contenders
SolidJS: Ultra-Fine-Grained Reactivity
SolidJS represents a fresh take on reactive JavaScript frameworks, combining React-like syntax with a powerful compilation model and an innovative approach to reactivity. SolidJS performs exceptionally well through true granular reactivity and compile-time optimisations, unlike traditional Virtual DOM frameworks.
The Reactive Revolution
At its core, SolidJS’s reactivity system is what sets it apart. Instead of using a Virtual DOM or relying on component-level updates, SolidJS tracks dependencies at a highly granular level. This means:
- Updates only affect the precise DOM elements that need to change
- No unnecessary re-renders or diffing operations
- Predictable and efficient performance at scale
- Minimal runtime overhead
Signals: The Building Blocks
SolidJS uses signals as its primary reactive primitive. Here’s a simple example:
import { createSignal } from 'solid-js'
function Counter() {
const [count, setCount] = createSignal(0)
return (
<button onClick={() => setCount(count() + 1)}>
Count: {count()}
</button>
)
}
Unlike React’s useState, signals are more than just state containers - they’re direct connections between your data and the DOM. Only the exact DOM nodes using that signal update when a signal’s value changes.
The Compiler Advantage
Similar to Svelte, SolidJS leverages compilation to optimise your code. During the build process, SolidJS:
- Transforms JSX into highly optimised DOM operations
- Eliminates dead code and unnecessary abstractions
- Produces minimal runtime code
- Generates efficient update mechanisms
Rich Reactive Primitives
SolidJS provides a comprehensive set of reactive primitives:
createEffect
import { createSignal, createEffect } from 'solid-js'
function Logger() {
const [count, setCount] = createSignal(0)
createEffect(() => {
console.log("Count changed:", count())
})
return <button onClick={() => setCount(c => c + 1)}>Increment</button>
}
createMemo
import { createSignal, createMemo } from 'solid-js'
function Expensive() {
const [number, setNumber] = createSignal(0)
const doubled = createMemo(() => {
// Only recalculates when number changes
return number() * 2
})
return <div>Double: {doubled()}</div>
}
Latest Features and Improvements
Recent versions of SolidJS have introduced several powerful capabilities:
Resource Management
SolidJS provides built-in tools for handling async data:
import { createResource } from 'solid-js'
function UserProfile({ userId }) {
const [user] = createResource(() => fetchUser(userId))
return (
<Show when={user()} fallback={<Loading />}>
<div>{user().name}</div>
</Show>
)
}
Control Flow Components
SolidJS includes efficient control flow components that maintain reactivity:
<Show>
for conditional rendering<For>
for list rendering<Switch>
and<Match>
for multiple conditions<Dynamic>
for dynamic component rendering
Developer Experience
SolidJS prioritises developer happiness with:
- Excellent TypeScript support out of the box
- Comprehensive DevTools for debugging
- Fast refresh during development
- Clear error messages and debugging
- A growing ecosystem of tools and libraries
Svelte: The Compiler-First Framework
Svelte takes a revolutionary approach to frontend development, setting it apart from frameworks like React and Vue. Instead of shipping a runtime library and relying on a Virtual DOM, Svelte shifts the heavy lifting to compile time, transforming your components into highly optimised vanilla JavaScript when you build your app.
The Compiler Advantage
The genius of Svelte lies in its compilation model. While traditional frameworks do most of their work in the browser, Svelte handles complex tasks during the build process. This means:
- Zero framework runtime overhead
- Smaller bundle sizes
- Faster initial page loads
- Lower memory usage
- Better performance on mobile devices
Reactive by Design
Svelte’s reactivity system is elegant in its simplicity. Rather than using hooks or complex state management solutions, reactivity is baked directly into the language syntax. Here’s a simple example:
<script>
let count = 0
function increment() {
count += 1
}
</script>
<button on:click={increment}>
Count: {count}
</button>
The compiler automatically tracks dependencies and updates the DOM precisely where needed, without the overhead of a Virtual DOM. This makes Svelte code not just performant, but also more intuitive to write and maintain.
What’s New in Svelte 5
The latest version of Svelte brings several game-changing features:
Runes: A New Take on Reactivity
Svelte 5 introduces “Runes”, a powerful new way to handle reactivity. Here’s how they work:
<script>
let count = $state(0)
function increment() {
count += 1
}
</script>
<button on:click={increment}>
Count: {count}
</button>
Runes offer more granular control over reactivity while maintaining Svelte’s signature simplicity. They’re perfect for component-local state management and provide a more functional approach to handling reactive values.
Cross-Environment Capabilities
With the introduction of Runnables, Svelte 5 breaks free from the browser. Components can now run in various environments:
- Server-side
- Web Workers
- Command-line scripts
- Edge computing environments
This flexibility makes Svelte an even more versatile choice for modern web applications.
Developer Experience Improvements
Svelte 5 doubles down on developer happiness with:
- Enhanced TypeScript support with better type inference
- Faster Hot Module Replacement (HMR)
- More intuitive error reporting
- Refined debugging tools
- Improved CSS scoping and style isolation
Partial Hydration
For applications prioritising performance, Svelte 5’s partial hydration feature allows developers to hydrate only a page’s interactive parts selectively. This means static content stays static, reducing unnecessary JavaScript processing and improving initial load times.
Astro: The All-in-One Web Framework
Astro represents a paradigm shift in web development, introducing an innovative approach combining static site generation with dynamic client-side interactivity. At its core, Astro is built around a revolutionary principle: ship zero JavaScript by default and add interactivity only where needed.
The Islands Architecture
Astro pioneered the “Islands Architecture,” a groundbreaking approach to web development that fundamentally changes how we think about page interactivity:
- Static HTML is rendered by default
- JavaScript is only loaded for interactive components
- Each interactive component is an “island” in a sea of static HTML
- Independent hydration of components based on their requirements
Here’s how an Astro island works:
---
// MyComponent.astro
import ReactCounter from '../components/ReactCounter'
import SvelteTimer from '../components/SvelteTimer'
---
<div class="page">
<!-- Static HTML -->
<header>
<h1>Welcome to my site</h1>
</header>
<!-- Interactive React component -->
<ReactCounter client:visible />
<!-- Interactive Svelte component -->
<SvelteTimer client:idle />
<!-- Static footer -->
<footer>
<p>© 2024 My Website</p>
</footer>
</div>
Framework Agnostic by Design
One of Astro’s most powerful features is its ability to work with multiple frameworks simultaneously. You can:
- Use React, Vue, Svelte, SolidJS, or Preact components
- Mix and match frameworks on the same page
- Leverage your existing component knowledge
- Choose the best tool for each specific use case
Client Directives: Fine-Tuned Hydration
Astro provides precise control over component hydration through client directives:
<!-- Different hydration strategies -->
<Component client:load /> <!-- Hydrate immediately -->
<Component client:idle /> <!-- Hydrate during idle time -->
<Component client:visible /> <!-- Hydrate when visible -->
<Component client:media="(max-width: 50em)" /> <!-- Hydrate based on media query -->
<Component client:only="react" /> <!-- Client-side only rendering -->
Content Collections
Astro’s content collections provide a robust system for managing content with type safety and enhanced developer experience. They act as a lightweight CMS built directly into your project:
// content/config.ts
import { defineCollection, z } from 'astro:content'
const blog = defineCollection({
schema: z.object({
title: z.string(),
publishDate: z.date(),
author: z.string(),
featured: z.boolean().default(false),
image: z.object({
src: z.string(),
alt: z.string()
}).optional(),
tags: z.array(z.string())
})
})
const products = defineCollection({
type: 'data', // JSON/YAML/CSV files
schema: z.object({
name: z.string(),
price: z.number(),
description: z.string(),
inStock: z.boolean()
})
})
export const collections = { blog, products }
Working with collections is straightforward and type-safe:
---
// pages/blog.astro
import { getCollection } from 'astro:content'
// All queries are fully typed!
const posts = await getCollection('blog', ({ data }) => {
return data.featured === true
})
// Individual entries are also typed
const recentPosts = posts
.sort((a, b) => b.data.publishDate.valueOf() - a.data.publishDate.valueOf())
.slice(0, 3)
---
<Layout>
{recentPosts.map(post => (
<article>
<h2>{post.data.title}</h2>
<time>{post.data.publishDate.toLocaleDateString()}</time>
{post.data.image && (
<img src={post.data.image.src} alt={post.data.image.alt} />
)}
</article>
))}
</Layout>
Performance by Default
Astro’s performance-first approach manifests in several key features:
- Zero JavaScript baseline
- Automatic page pre-rendering
- Efficient asset optimisation
- Smart image handling
- Minimal client-side hydration
Zero-JS Pattern
---
// Regular components ship zero JavaScript
const items = ["Item 1", "Item 2", "Item 3"]
---
<ul>
{items.map(item => <li>{item}</li>)}
</ul>
Asset Optimisation
Astro automatically handles asset optimisation with features like:
---
import { Image } from 'astro:assets'
---
<!-- Images are automatically optimised -->
<Image
src={import('../assets/hero.png')}
width={800}
height={400}
format="avif"
alt="Hero image"
/>
<!-- CSS is automatically minified and bundled -->
<style>
img {
border-radius: 0.5rem
box-shadow: 0 4px 6px -1px rgb(0 0 0 / 0.1)
}
</style>
Partial Hydration Example
---
---
<Layout>
<!-- Static Header -->
<header>
<h1>Welcome to my store</h1>
</header>
<!-- Interactive shopping cart only loads when visible -->
<ShoppingCart client:visible>
{items.map(item => (
<CartItem
name={item.name}
price={item.price}
client:visible
/>
))}
</ShoppingCart>
<!-- Product grid remains static -->
<ProductGrid products={products} />
</Layout>
Server-Side Rendering (SSR)
With SSR mode, Astro offers dynamic server rendering when needed:
---
// pages/data.astro
if (!Astro.cookies.has('session')) {
return Astro.redirect('/login')
}
const data = await fetchDataFromAPI()
---
<Layout>
<h1>Dynamic Data</h1>
{data.map(item => <DataCard item={item} />)}
</Layout>
Latest Features and Improvements
Recent versions of Astro have introduced several powerful capabilities:
View Transitions API
---
import { ViewTransitions } from 'astro:transitions'
---
<html>
<head>
<ViewTransitions />
</head>
<body>
<main transition:animate="slide">
<slot />
</main>
</body>
</html>
Middleware Support
// middleware.ts
export function onRequest({ request, redirect }, next) {
const token = request.headers.get('authorization')
if (!token) {
return redirect('/login')
}
return next()
}
Developer Experience
Astro prioritises developer productivity with:
- Excellent TypeScript support
- Fast development server with HMR
- Comprehensive documentation
- Strong community and ecosystem
- Built-in markdown support
- Intuitive project structure
- Powerful CLI tools
Astro’s developer experience goes beyond just tools - it’s built into the framework’s DNA:
Type Safety Throughout
// src/env.d.ts
/// <reference types="astro/client" />
interface ImportMetaEnv {
readonly DATABASE_URL: string
readonly API_KEY: string
}
// Now environment variables are typed
const db = new Database(import.meta.env.DATABASE_URL)
Advanced Routing Patterns
typescriptCopy// pages/blog/[...slug].astro
export async function getStaticPaths() {
const posts = await getCollection('blog')
return posts.map(post => ({
params: { slug: post.slug },
props: { post },
}))
}
// Dynamic route with type-safe params
export async function getStaticPaths({ paginate }) {
const allPosts = await getCollection('blog')
return paginate(allPosts, { pageSize: 10 })
}
Dev Server Features
The development server includes:
- Hot Module Replacement (HMR) that maintains state
- Automatic error overlay with stack traces
- Network condition simulation
- Command palette for quick actions
Integration Ecosystem
Astro’s integration system makes it easy to add functionality:
- Framework integrations (React, Vue, Svelte, etc.)
- Styling tools (Tailwind, Sass, etc.)
- Performance optimisations (Image tools, minification)
- CMS connectors (Contentful, Sanity, etc.)
- Authentication providers
- Deployment platforms
Astro’s integration system is both powerful and flexible, allowing you to extend functionality while maintaining performance:
Framework Components
---
// Mix multiple frameworks in one file
import ReactCounter from '../components/ReactCounter'
import SvelteTimer from '../components/SvelteTimer'
import VueHeader from '../components/VueHeader'
---
<VueHeader client:load>
<ReactCounter client:visible />
<SvelteTimer client:idle />
</VueHeader>
Advanced Integrations Example
// astro.config.mjs
import { defineConfig } from 'astro/config'
import react from '@astrojs/react'
import tailwind from '@astrojs/tailwind'
import vercel from '@astrojs/vercel/serverless'
export default defineConfig({
integrations: [
react(),
tailwind({
// Custom Tailwind configuration
config: { darkMode: 'class' }
})
],
output: 'server',
adapter: vercel({
analytics: true,
imageService: true,
webAnalytics: {
enabled: true
}
})
})
Key Features Comparison
Syntax and Learning Curve
Modern web development frameworks each take their own unique approach to solving similar problems. While SolidJS, Svelte, and Astro all aim to make web development more efficient, their syntactical approaches and learning curves vary significantly, reflecting different philosophies about how web applications should be built.
SolidJS: Familiar Territory with New Concepts
// SolidJS Component Example
import { createSignal } from "solid-js"
function Counter() {
const [count, setCount] = createSignal(0)
return <button onClick={() => setCount(count() + 1)}>Clicks: {count()}</button>
}
At first glance, SolidJS is closely aligned with React’s methodology, using JSX syntax and functional components. This familiar surface-level syntax makes it an appealing choice for React developers looking to transition to a more performance-oriented framework. However, beneath this familiar exterior lies a fundamentally different reactive system.
The framework’s signal-based reactivity system represents a significant departure from React’s virtual DOM approach. Instead of relying on component re-renders, SolidJS uses fine-grained reactivity to update only the specific DOM elements that need to change. While more efficient, this system requires developers to shift their mental model of how state updates propagate through an application.
For developers coming from a React background, the learning curve can be steep despite the syntactic similarities. The need to call signals as functions (e.g., count()
) rather than accessing them as variables takes time to internalise. Additionally, the absence of component re-renders means many familiar React patterns must be re-imagined.
Key learning challenges include:
- Understanding signal creation and usage
- Adapting to fine-grained reactivity patterns
- Learning when to use reactive primitives effectively
- Mastering SolidJS’s unique optimisation techniques
Svelte: Embracing Simplicity
<!-- Svelte Component Example -->
<script>
let count = 0
</script>
<button on:click={() => count += 1}>
Clicks: {count}
</button>
Svelte takes a radically different approach by prioritising developer experience through simplicity. The framework’s syntax feels natural and intuitive, closely resembling vanilla JavaScript and HTML. This approach has made Svelte particularly appealing to developers new to frontend frameworks and those fatigued by the complexity of other modern frameworks.
The compiler-first approach of Svelte eliminates the need for a runtime library, transforming your components into highly optimised vanilla JavaScript during the build process. This results in smaller bundle sizes and simplifies the mental model required to understand how your application works.
Svelte’s reactive system is its most elegant feature. Unlike SolidJS’s signals or React’s useState, Svelte allows developers to trigger reactivity through simple variable assignments. This feels more natural and requires less boilerplate code, though it has its own set of rules to learn.
The framework’s built-in features for handling common web development tasks - such as scoped styling, animations, and state management - are thoughtfully designed and well-integrated. This comprehensive approach means developers spend less time configuring third-party solutions and more time building features.
Astro: Content-First Development
---
// Astro Component Example
let count = 0
---
<button id="counter">
Clicks: {count}
</button>
<script>
const button = document.getElementById("counter")
let count = 0
button.addEventListener("click", () => {
count++
button.textContent = `Clicks: ${count}`
})
</script>
Astro represents a paradigm shift in how we think about web application architecture. Its content-first approach and unique component format reflect a growing understanding that not every element on a page needs to be interactive by default.
The framework’s component structure cleanly separates concerns through its distinctive frontmatter format. Server-side logic lives between the frontmatter fences (---), while the template syntax below handles presentation. This separation makes it easier to reason about where code will execute and how it will affect the user experience.
What truly sets Astro apart is its partial hydration system, often referred to as the “Islands Architecture.” This approach allows developers to selectively hydrate components, ensuring that JavaScript is only loaded where it’s actually needed. While this can lead to significant performance improvements, developers must carefully consider their application’s interactivity needs.
Development Environment and Tooling
The development experience varies significantly across these frameworks. Svelte offers the smoothest initial setup, with its well-documented template and minimal configuration requirements. The framework’s excellent error messages and comprehensive documentation make the development process more approachable for newcomers.
SolidJS, while requiring more initial setup, provides robust tooling support and excellent TypeScript integration. This makes it particularly suitable for larger, more complex applications where type safety and developer tooling are crucial.
Astro balances these approaches, offering comprehensive starter templates while maintaining the flexibility to handle more complex configurations when needed. Its build system is particularly powerful, though mastering its various optimisation options takes time.
Making the Choice
When choosing between these frameworks, consider your team’s background and your project’s requirements:
- For teams with React experience looking for better performance: SolidJS offers a familiar syntax with superior runtime characteristics
- For new projects prioritising developer experience: Svelte’s intuitive syntax and comprehensive features make it an excellent choice
- For content-heavy sites with selective interactivity: Astro’s partial hydration approach can provide the best of both worlds
The learning curve for each framework ultimately depends on your starting point and your project’s needs. Svelte generally offers the gentlest learning curve, while SolidJS and Astro require more upfront investment to master their unique concepts. However, all three frameworks provide well-thought-out solutions to modern web development challenges, each with its strengths and trade-offs.
Performance and Build Size
Performance in modern web frameworks isn’t just about runtime speed—it encompasses bundle size, initial load time, time-to-interactive, and overall runtime efficiency. Each of our featured frameworks takes a distinct approach to these challenges, with impressive results across different metrics.
SolidJS has made remarkable strides in minimising bundle size without sacrificing functionality. It consistently produces some of the smallest bundles in the industry for single-page applications, often coming in under 10KB for a basic application. This achievement stems from its unique reactive system that eliminates the need for a virtual DOM, instead using compiled templates that update the DOM directly. The result is not just a tiny bundle size but also exceptional runtime performance that often outperforms other frameworks in benchmarks.
Svelte takes a different approach to performance optimisation through its compile-time framework strategy. Instead of shipping a runtime library, Svelte compiles your components into highly optimised vanilla JavaScript during the build process. This compilation step generates precisely the JavaScript needed for your components to function, eliminating unnecessary overhead. The generated code is highly efficient, while bundle sizes may be slightly larger than those of SolidJS for basic applications. It requires minimal runtime overhead, leading to excellent performance, especially in interaction-heavy applications.
Astro’s performance story centres around its “zero JavaScript by default” philosophy, which has profound implications for web performance. By shipping only the necessary JavaScript for interactive components, Astro sites typically achieve incredible performance metrics out of the box:
- Faster page loads due to minimal initial JavaScript
- Better performance on mobile devices
- Improved core web vitals scores
- Reduced bandwidth usage
This approach particularly shines for content-focused websites where much of the interactivity is optional or limited to specific components.
Server-Side Rendering and Static Site Generation
Modern web applications demand flexible rendering strategies to meet diverse performance and SEO requirements. Each framework has evolved to address these needs, though their approaches and strengths differ significantly.
SolidJS: Versatile Rendering Options
SolidJS has invested heavily in server-side rendering capabilities, offering both traditional SSR and streaming SSR. The streaming SSR capability is particularly noteworthy, allowing applications to send content to the client progressively as it becomes available. This can significantly improve perceived performance, especially for data-heavy pages.
// SolidJS SSR Example
export default function ProductPage(props) {
const [product] = createResource(() => fetchProduct(props.id))
return (
<Suspense fallback={<LoadingSpinner />}>
<ProductDetails product={product()} />
</Suspense>
)
}
The framework’s fine-grained reactivity system works seamlessly with its SSR implementation, ensuring efficient hydration and optimal performance after the initial render.
Svelte: The SvelteKit Advantage
Svelte’s server-side rendering story is primarily told through SvelteKit, its official application framework. SvelteKit provides a robust platform for building applications with various rendering strategies:
- Server-side rendering with efficient hydration
- Static site generation for content-heavy sites
- Hybrid rendering approaches that combine both strategies
<!-- SvelteKit SSR Example -->
<script>
/** @type {import('./$types').PageData} */
export let data
</script>
<h1>{data.title}</h1>
<article>{@html data.content}</article>
SvelteKit’s approach to SSR is particularly developer-friendly, with intelligent defaults that make it easy to build performant applications without extensive configuration. The framework automatically optimises the hydration process, sending only the JavaScript necessary for interactivity.
Astro: Static-First with Dynamic Capabilities
Astro’s approach to rendering reflects its content-first philosophy. While it excels at static site generation, its partial hydration system (Islands Architecture) provides flexible options for adding dynamic content:
---
// Astro Static Generation with Dynamic Island
import InteractiveCounter from '../components/Counter.jsx'
---
<article>
<h1>Static Content</h1>
<p>This content is generated at build time.</p>
<InteractiveCounter client:visible />
</article>
The client:*
directives provide fine-grained control over component hydration, allowing developers to specify exactly when and how components become interactive. This approach offers several benefits:
- Optimal performance for static content
- Selective hydration for interactive components
- Flexible loading strategies for different use cases
Community and Ecosystem Support
A framework’s community and ecosystem can be as important as its technical merits regarding real-world development. The landscape of community support and available resources varies significantly among these frameworks.
Svelte has cultivated the most mature ecosystem of the three, with a vibrant community that has produced an extensive collection of components and tools. The framework’s popularity has led to:
- Comprehensive documentation and tutorials
- Regular community events and conferences
- A wide range of third-party components and libraries
- Strong integration with popular development tools
This mature ecosystem makes Svelte an attractive choice for production applications, as developers can often find pre-built solutions for common requirements.
Astro’s community has seen remarkable growth since its introduction, particularly among developers building content-focused websites. The framework has attracted strong support from:
- Content management platforms
- Static site hosting providers
- Documentation tool developers
- Technical content creators
This focused growth has resulted in an ecosystem particularly well-suited for content-heavy applications, with excellent tools and integrations for managing and deploying content-focused sites.
SolidJS has fostered a highly engaged and technically sophisticated user base while having a smaller community. The community’s focus on performance-critical applications has led to:
- High-quality, performance-oriented components
- Advanced tooling for optimisation
- Deep technical discussions and knowledge sharing
- Strong TypeScript integration and type support
While the ecosystem may be smaller than Svelte’s, available resources’ quality and performance focus make it particularly valuable for teams building performance-critical applications.
The choice between these frameworks often comes down to ecosystem needs:
- Choose Svelte when you need a wide range of battle-tested components and tools
- Choose Astro when building content-focused sites with specific integration needs
- Choose SolidJS when performance is critical, and you’re willing to build more custom solutions
Each community continues to grow and evolve, but understanding their current state and trajectory can help inform framework selection for your next project.
Making the Right Choice for Your Project
After diving deep into these frameworks’ technical aspects, performance characteristics, and community support, the natural question arises: which one should you choose for your next project? While each framework offers compelling advantages, the decision ultimately depends on your specific context and requirements.
Understanding Framework Sweet Spots
SolidJS: The Performance Powerhouse
SolidJS truly shines in scenarios where performance and precision are paramount. Its fine-grained reactivity system makes it the ideal choice for:
Data-intensive applications where real-time updates are crucial, such as:
- Financial dashboards with live data streams
- Complex data visualisation tools
- Real-time collaboration platforms
The framework’s minimal bundle size and exceptional runtime performance make it particularly well-suited for applications where every millisecond counts. However, this power comes with the trade-off of a steeper learning curve and a less mature ecosystem. Teams choosing SolidJS should be prepared to invest in building custom solutions and maintaining more of their own infrastructure.
Svelte: The Developer’s Delight
Svelte has earned its reputation as one of the most developer-friendly frameworks in the ecosystem. Its intuitive syntax and comprehensive feature set make it an excellent choice for:
- Teams transitioning from traditional web development
- Projects requiring rapid development and iteration
- Applications needing built-in animation and state management
The framework’s mature ecosystem and excellent documentation mean you’ll spend less time-solving common problems and more time building unique features for your application. Svelte excels in traditional web applications where developer productivity and maintainability are key priorities.
Astro: The Content Champion
Astro has revolutionised how we think about content-first web development. It’s the natural choice for:
- Content-rich websites like blogs and documentation
- Marketing sites requiring excellent SEO
- Multi-framework projects requiring gradual migration
Its unique partial hydration approach ensures optimal performance while maintaining the flexibility to add interactivity where needed. The framework’s ability to seamlessly integrate multiple frontend technologies makes it an excellent choice for larger organisations with diverse technical requirements.
Strategic Framework Selection
When guiding our clients at tpsTech through framework selection, we consider several critical factors that impact long-term project success:
Technical Considerations
- Performance Requirements: How critical is raw performance versus developer productivity?
- Scale and Complexity: Will the application need to handle complex state management and data flow?
- SEO and Initial Load Time: How important is search engine optimisation and first-page load?
Team and Organisation Factors
- Team Expertise: What is your team’s current technical background and learning capacity?
- Development Timeline: How quickly must the project be completed?
- Long-term Maintenance: Who will maintain the application, and what are their capabilities?
Business Impact
- Time to Market: How quickly do you need to launch and iterate?
- Budget Constraints: What resources are available for development and maintenance?
- Growth Projections: How might your needs evolve as your business grows?
Professional Guidance and Support
At tpsTech, we understand that choosing a framework is only the beginning of your web development journey. Our experienced team offers comprehensive support throughout the entire process:
Assessment and Planning
- Technical requirements analysis
- Team capability evaluation
- Performance benchmarking
- Scalability planning
Implementation and Development
- Architecture design
- Component development
- Performance optimisation
- Testing and quality assurance
Ongoing Support
- Maintenance and updates
- Performance monitoring
- Team training and support
- Technical consultation
Conclusion: A Framework for Every Need
The modern web development landscape offers powerful tools for every use case. SolidJS brings unprecedented performance and precision for demanding applications. Svelte offers an excellent balance of developer experience and capability. Astro provides an innovative solution for content-first development with selective interactivity.
The key to success lies in choosing a framework and understanding how to leverage its strengths for your specific needs. Whether you’re building a high-performance web application, a content-rich marketing site, or anything in between, contact us to discuss how we can help you make the most of these powerful tools.
By carefully considering your project’s requirements, team capabilities, and long-term goals, you can make an informed decision that sets your project up for success. Our team at tpsTech is here to help you navigate these choices and build exceptional web experiences that serve your business needs.
Need Help With Your Web Development Project?
Choosing the right framework is crucial for your project’s success. Our team at tpsTech has extensive experience with modern web frameworks. It can help you make the right choice for your specific needs. We offer comprehensive web development services that ensure your project is built with the most suitable technology stack.
Whether you’re starting a new project or looking to optimise an existing one, our experts can guide you through the process and implement the best solution for your business. Contact us to discuss your project requirements and how we can help you achieve your web development goals.
[!NOTE] Framework comparisons and performance metrics are based on current versions as of January 2024. Always check the latest documentation and benchmarks when making your decision.