Beginners Guide to Writing Clean Maintainable Code
Learn clean code principles with practical examples. Improve code quality, reduce technical debt, and write maintainable, efficient code for web development.

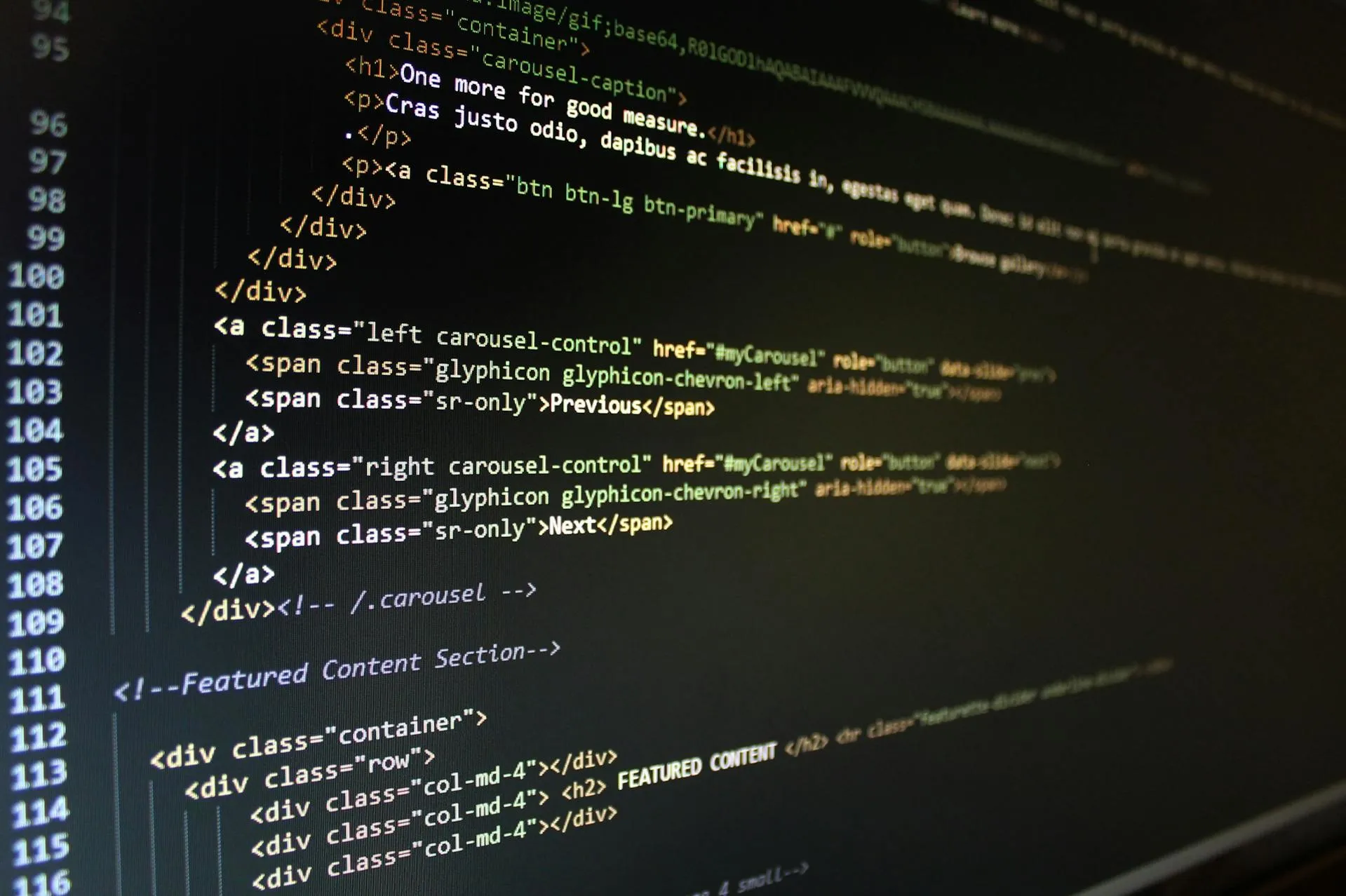
Table of Contents
- Table of Contents
- Introduction
- Understanding Clean Code
- Essential Clean Code Principles
- Writing Readable Code
- Common Coding Mistakes
- Clean Code Examples
- Tools for Maintaining Clean Code
Introduction
Writing clean code is fundamental to successful web development. Clean code, which refers to code that is easy to understand, maintain, and modify, is not just about making your code work—it’s about making it work well for both computers and humans. As professional web developers at tpsTech, we understand that clean code is essential for creating sustainable and scalable web solutions.
Understanding Clean Code
Clean code is more than just proper formatting—it’s a comprehensive approach to writing code that others (including your future self) can easily understand and maintain. Let’s break down the key aspects:
Code Readability: Code readability means writing code that clearly expresses its purpose without requiring extensive documentation. Think of it like writing a story—each line should flow logically into the next, making the overall narrative (functionality) clear to anyone reading it.
Code Maintainability: Maintainable code is code that can be easily modified or extended without introducing bugs. This is crucial for web development projects that evolve over time. When code is maintainable, making changes or fixing issues becomes significantly easier and less time-consuming.
Technical Debt: Technical debt is a term that describes the future cost (in time and resources) of choosing an easy solution now instead of using a better approach that would take longer to implement. Clean code helps minimise technical debt by creating a solid foundation that’s easier to build upon.
Essential Clean Code Principles
Let’s explore the fundamental principles of clean code:
Meaningful Names: Variable and function names should clearly describe their purpose. For example:
// Poor naming
const x = 5 // What does x represent?
// Clean naming
const maxLoginAttempts = 5 // Clear purpose
Single Responsibility: Each function or class should do one thing and do it well. This principle, known as the Single Responsibility Principle (SRP), makes code easier to test and maintain:
// Multiple responsibilities (avoid)
function validateAndSaveUser(userData) {
// Validation logic
// Database operations
// Email notifications
}
// Single responsibility (preferred)
function validateUserData(userData) {
// Only handles validation
}
function saveUser(validatedData) {
// Only handles saving
}
function notifyUser(userId) {
// Only handles notifications
}
DRY (Don’t Repeat Yourself): The DRY principle encourages code reuse and helps prevent inconsistencies. Here’s an example:
// Repetitive code (avoid)
function calculateGSTInclusive(price) {
return price + price * 0.1
}
function calculateGSTExclusive(priceWithGST) {
return priceWithGST - priceWithGST * 0.1
}
// Clean, DRY code
const GST_RATE = 0.1
function calculateGST(amount, inclusive = true) {
return inclusive ? amount + amount * GST_RATE : amount - amount * GST_RATE
}
Writing Readable Code
Readable code follows consistent patterns and formatting. Here are key practices:
Consistent Formatting: Use consistent indentation, spacing, and line breaks. Most modern editors support automatic formatting tools. At tpsTech, we recommend using tools like Prettier or ESLint to maintain consistency:
// Poor formatting
function calculateTotal(items) {
for (let i = 0; i < items.length; i++) {
total += items[i].price
}
}
// Clean formatting
function calculateTotal(items) {
let total = 0
for (let i = 0; i < items.length; i++) {
total += items[i].price
}
return total
}
Comments and Documentation: Write comments that explain why something is done, not what is done. The code itself should be clear enough to explain what it does:
// Poor commenting
// Loop through array
for (let i = 0; i < items.length; i++) { ... }
// Good commenting
// Calculate running total excluding cancelled orders
for (let i = 0; i < items.length; i++) { ... }
Common Coding Mistakes
Let’s examine common mistakes that lead to unclear code:
Deep Nesting: Deeply nested code is hard to follow and maintain. Use early returns and guard clauses to reduce nesting:
// Deep nesting (avoid)
function processOrder(order) {
if (order) {
if (order.items) {
if (order.items.length > 0) {
// Process order
}
}
}
}
// Clean code with guard clauses
function processOrder(order) {
if (!order) return
if (!order.items?.length) return
// Process order
}
Magic Numbers: Avoid using unexplained numbers in your code. Use constants with meaningful names:
// Magic numbers (avoid)
if (user.age >= 18) { ... }
// Clean code with constants
const LEGAL_ADULT_AGE = 18;
if (user.age >= LEGAL_ADULT_AGE) { ... }
Clean Code Examples
Let’s look at practical examples across different web technologies:
HTML Example:
<!-- Poor HTML structure -->
<div>
<div>
<div class="btn">Click me</div>
</div>
</div>
<!-- Clean HTML structure -->
<main class="content">
<section class="product-section">
<button class="product-button">Click me</button>
</section>
</main>
CSS Example:
/* Poor CSS organisation */
.button {
background: blue;
}
.button2 {
background: blue;
}
/* Clean CSS with BEM methodology */
.button {
background-color: var(--primary-color);
padding: var(--spacing-medium);
}
.button--primary {
background-color: var(--primary-color);
}
.button--secondary {
background-color: var(--secondary-color);
}
Tools for Maintaining Clean Code
Several tools can help maintain clean code standards:
Code Formatters:
- Prettier: Automatically formats your code
- ESLint: Identifies and fixes code quality issues
- StyleLint: Ensures consistent CSS/SCSS formatting
Version Control: Using Git with clear commit messages helps track code changes and maintain code quality. At tpsTech, we follow conventional commit messages for clarity:
# Poor commit message
git commit -m "fixed stuff"
# Clean commit message
git commit -m "fix: correct validation logic in user registration"
Clean code is an investment in your project’s future. While it may take more time initially, it saves significant time and resources in the long run. As your web development partner, tpsTech can help you implement these clean code practices in your projects.
Need help implementing clean code practices in your web development project? Contact our team for professional development services that prioritise code quality and maintainability.